This is the sixth in a series of blog posts I’ve been working on during COVID isolation. It started with the idea of refreshing my systems design and software engineering skills, and grew in the making of it.
Part 1 describes ‘the problem’. A mathematics game designed to help children understand factors and limits which represents the board game Ludo.
Part 2 takes you through the process I followed to break this problem down to understand the constraints and rules that players follow as a precursor to implementation design.
Part 3 shows how the building blocks for the design of the classes and methods are laid out in preparation for understanding each of the functions and attributes needed to encapsulate the game.
In Part 4 we get down to business… producing the game using the programming language PHP with an object orientated approach.
Part 5 stepped it up a notch and walked through a translation of the code to object orientated C++.
Now in Part 6 I do the same thing, but in the world of Java.
Get in touch on Twitter at @mr_al if you’d like to chat.
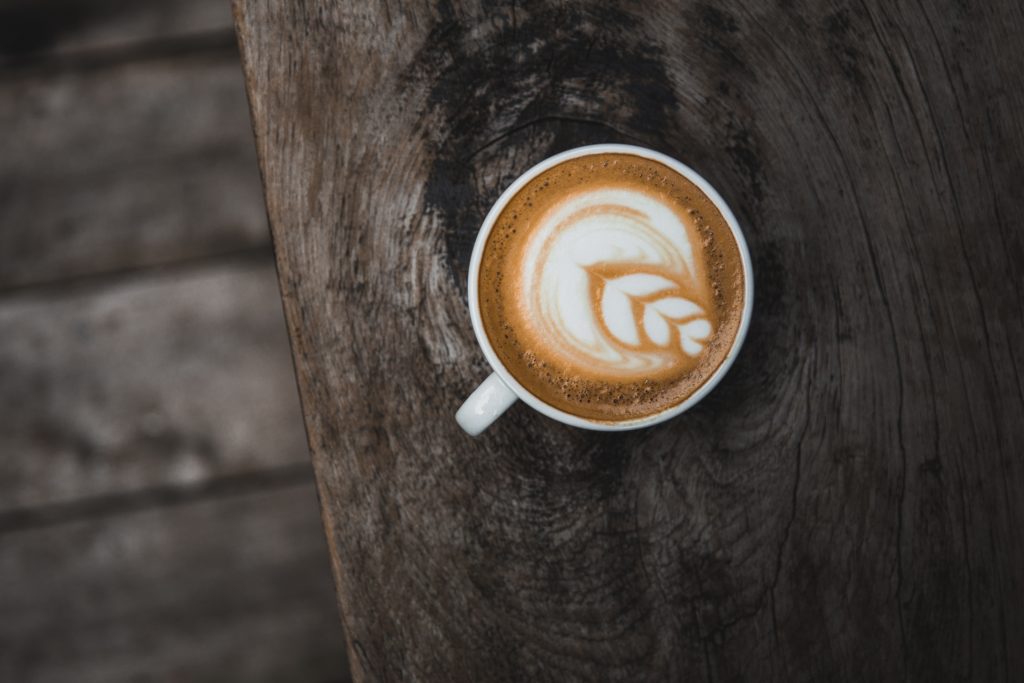
Java
Available on GitHub at: https://github.com/mr-alistair/ootest-java
I’ve never officially been ‘taught’ Java. I missed out on that from a University perspective by a few years and was only ever exposed to the compiled after-math in the ‘90s and ‘00s.
Overcoming prejudice
My feelings about Java have been mixed. Early exposure in the corporate world was to chunky Windows-GUI analogues that didn’t look as polished a their C++ or VB peers, leaky unix-based apps that required regular garbage-dumps that would freeze activity system-wide while they took out their memory-trash, and intimidating thread dumps that provided wholly unsatisfying evidence as to what had actually gone wrong, and why.
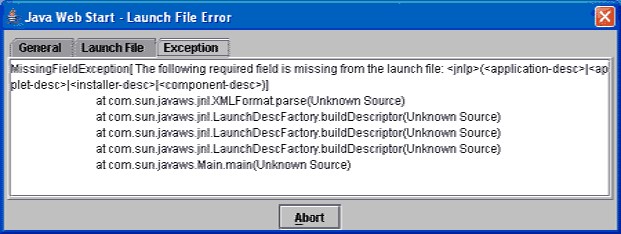
In terms of technical prejudice, my inner sceptic had become wary of any solution that positions itself as being pan-platform, write-once-run-anywhere, saviour of all developers, etc. The hype of so-called ‘5th General Languages’ (5GLs) in the ‘80s had also made me wary. The reality of having to specifically match a range of different Java Runtime libraries based on the vendor, platform and operating system simply meant that did not live up to the hype. (I was particularly scarred by trying to get an enterprise integration platform up and running in a Production environment, and the darn thing just would…not…start. Turned out there was a minor (I’m talking x.x.00n) version difference in the Java runtime libraries between the non-prod and prod environments, and that was all it took.)
Oh, and the typeface on any GUI implementation always left me cold… felt like I was back in the 16-bit graphics days. Nostalgia will only get you so far.
Forearmed
Armed with my knowledge of what was different between PHP and C++, I had a better idea of what I would face in converting the algorithm to Java. My first move, however, was to look for an appropriate IDE. I tried in vain to find suitable project plugins for Visual Studio which matched the toolkit I’d used when coding in C++. Having previously attempted to code Android Java in Eclipse, I downloaded that instead and got to work.
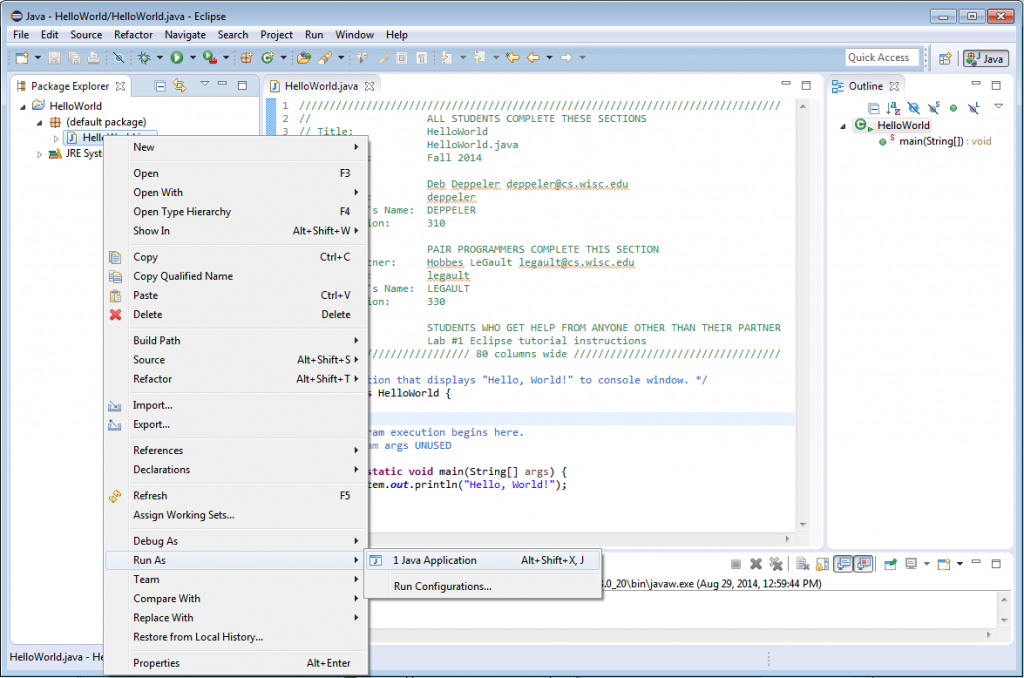
Translation from C++ to Java
The transcription was far easier than I though it would be. The syntax and grammar of the languages was a lot closer than anticipated, save for the nuances between how things are defined. It’s a bit like the difference between German-German, and Swiss-German. Close, but with klein aber fein differences.
Class definitions and internal references to properties did not require the use of ‘this’. Clearly it was self-evident to Java that ‘this is mine’ was the default and did not need to be explicitly mentioned.
As for Booleans, False was false and True was true. Easy.
Classy Arrays
Instantiating an array of another Class took me a few goes as I was over-complicating it. Java allowed the simple call of:
Marker[] p_pieces = new Marker[5];
…to tell the compiler ‘this property will be a 5-element array of class type Marker’. As a BASIC programmer from way back, it took me a little while to get used to having to define a property as a type of the Class you were about to tell it that it was, anyway. It was not helped by online articles and help-support topics that showed it like so:
Classname classname = new Classname;
(or, more frustratingly: Foo foo = new Foo; )
This always tripped me up… especially when the writer would give the property/variable name the same name as the Class. Took me a lot of trial and error (and stackoverflow trawling) to realise that there were a few things going on here. Simply declare the property, and you end up with a null property. Declare AND instantiate it at the same time, and you get a property that is enlivened with whatever the Constructor method for that Class provides.
Two dimensional arrays followed a similar path. In the Game class method g_target_magic_numbers there was a need to instantiate a two-dimensional array of integers. After some mucking about this ended up being simple:
int[][] x_forecast_pointers = new int[5][3];
What I realised earlier, though, was that the element values in this array needed to be set BEFORE they could be referenced, or a null pointer error was returned. So, for one-dimensional arrays where a value may or may not be filled, it was a case of saying up front:
int[] x_piece_array_pointer = {0,0,0,0,0};
Making a hash of it
Searching for the existence of a value in an array gave me the chance to try out a different method – hashing – which research had informed me was a more efficient over iterating across the entire array.
Establishing a hash set from an array of values involved including the following class libraries, and making the following calls:
import java.util.Arrays;
import java.util.HashSet;
final Set<Integer> x_temp_magic_numbers = new HashSet<Integer>();
x_temp_magic_numbers.addAll( Arrays.asList( 20,24,30,40,60));
So now we have a hash set named x_temp_magic_numbers that contains the five values.
Returning a Boolean when searching for a value within this hash set occurs through:
x_test_1 = x_temp_magic_numbers.contains(x_player.p_pieces[x_temp_value].m_get_location());
[vis, return a true/false IF the location of x_player’s piece number x_temp_value (which is identified through the method m_get_location) exists in the hash set held by x_temp_magic_numbers]
At this point, I was pining for PHP’s “in_array” method.
Do what I say, not what I intend
Copying arrays was also straightforward…but came with another hitch. Initially, I did the following:
System.arraycopy(x_piece_array_pointer, 0, x_piece_array_backup, 0, 5);
I’d tried to be economical (read: lazy) and ignore array position zero as I was only interested in the values in positions 1 through to 4 – the array of Markers for each Player. I even left myself a prompter note in the comments:
//hopefully won’t fail as index 0 is not set
A few hours later, this comment read:
//hopefully won’t fail as index 0 is not set //well…it did…
..because of exactly what I had predicted. In a Java array, even if you have happily populated 4 out of the 5 elements… if one element is null, then a method such as arraycopy will crack it. Also, the final integer passed to arraycopy is the total length of the array, not a pointer to the final index (which would typically be 4).
What arraycopy did do (when I corrected my issues of having null elements) was to create a true new instance of the array, rather than a pointer to the original one. This took me a while to work through, as if I’d simply said “this array [equals] that array” it would assume that I wanted a logical reference, not a fresh and independent copy.
Random variations
Getting an integer random number was fun. I used the java.util.Random library and generated it like so:
Random r = new Random();
return r.nextInt(g_upper) + 1;
The second line takes some explaining. Random() by itself sets a seed and initiates the random number generator. nextInt(value) generates an integer between 0 and the provided value (in this case identified by the variable ‘g_upper’)…minus 1. So, if you want a random number like a die roll, you need to get the method to add one to the value generated.
Java coded packages tied up with strings
Couple of final things in the Java world: strings and concatenation were easier to handle following my traumas with C++. The difference being the need to explicitly call the ‘toString’ method before the Integer class of the object, even though the variable object was an Integer. For example, in the logging method:
g_logmove(“Player ” + Integer.toString(g_playerturn) + ” will go first.“);
Formatting date/time in the logging method took a bit of tweaking as well. Rather than trying to squeeze it all into one line, I broke it up for readability.
Integer x_movecounter = this.g_movecounter;
DateTimeFormatter dtf = DateTimeFormatter.ofPattern(“yyyy-MMM-dd HH:mm:ss“);
LocalDateTime now = LocalDateTime.now();
String x_timestamp = dtf.format(now);
g_movelog[x_movecounter] = (x_timestamp + ” — ” + x_logmove);
Wrap up
Some interesting observations – Java did not suffer the same random-seed issue that I had experienced in C++. I was able to punch out to a command line and execute it from my workspace using:
java -classpath Auto120 Auto120.auto120
…which enabled me to capture some interesting stats on repeated runs which I’ll discuss in a later post.
And, indeed, I was able to pick up and run the code on an Ubuntu Linux installation with no modifications. Heh – turns out what is written on the side of the pack is true. Go figure.
Java was a bit of fun, and by now I was seeing a regular pattern in the things that differed (or aligned) between the languages. Therefore, translating from Java to Javascript should be pretty straight forward, right? Right…? Hello..? (taps microphone)
NEXT POST… Javascript. It’s not quite Java, and it’s not a pure scripting language. So what gives?