This is the first in a long series of blog posts I’ve been working on during COVID isolation. It started with the idea of refreshing my systems design and software engineering skills, and grew in the making of it.
I’m hoping this will interest and inspire others who are either starting out with their interest in design and coding, or who are curious to explore and learn more beyond what they currently understand.
So, here is Part 1. Get in touch on Twitter at @mr_al if you’d like to chat.
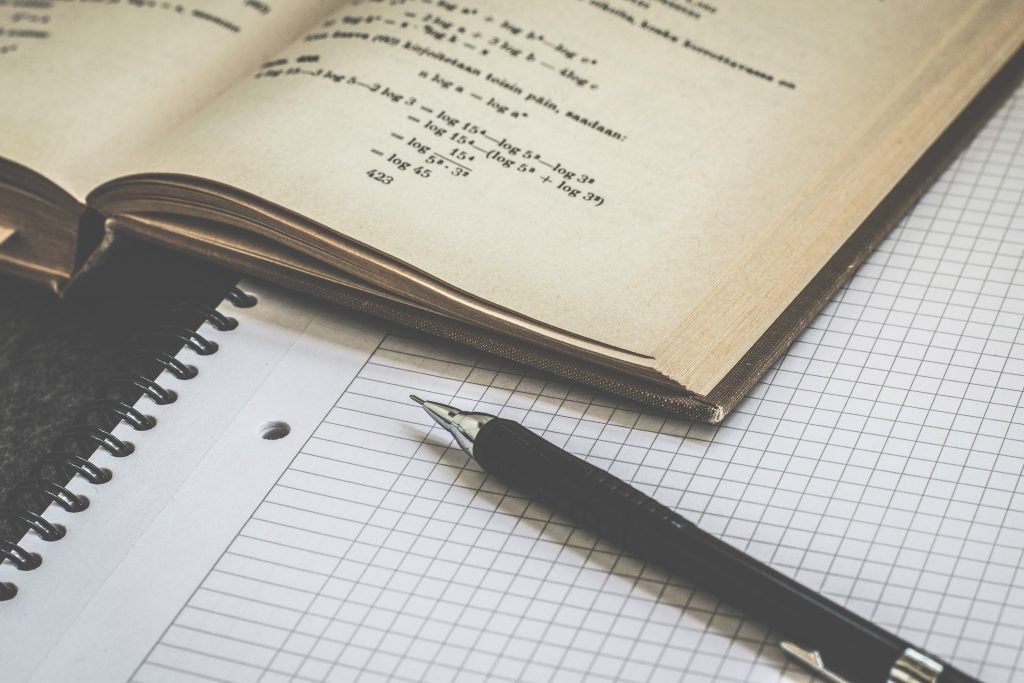
Auto120 – Description of problem
I decided to explore an algorithmic implementation of the “Multiplication Ludo” game as published by Dr. James Russo of Monash University, Australia.
Russo, James. (2018). Get your game on: Multiplication Ludo. (Prime Number: Volume 33, Number 3, 2018. The Mathematical Association of Victoria)
https://www.researchgate.net/publication/326571291_Get_your_game_on_Multiplication_Ludo
The original algorithm and rules derived from Dr. Russo’s description. Interpretation and rules sequencing by Alistair Lloyd (me!) May-June 2020. My awareness of this was through publication by Mr. Michael Minas, editor of Prime Number and award winning educator.
Set up:
• Two Players face a board with Positions marked from 1 to 120 inclusive
• Each Player has four Markers
• Every Marker starts at position 1
• The first Player to move all 4 of their Markers to land exactly on Position 120 is the winner
Game Play:
• A Player is chosen at random to go first
• Players take turns rolling a six-sided die
• If a Player roles a 1, they forfeit their turn
• If a Player roles a number between 2 and 6 inclusive, they can move one of their Markers
• The movement is calculated as the PRODUCT of [i] the die roll, and [ii] the value of the Position of the Marker that the Player choses to move
• (i.e. if the Player rolls a 5, and choses to move Marker 1 on Position 1, then they may move this Marker to Position 5 (5 x 1 = 5)
• (i.e. if the Player rolls a 4, and choses to move Marker 3 on Position 16, then they move this Marker to Position 64 (4 x 16 = 64)
• If the destination Position is already occupied by one or more of the Opposing Player’s Markers, then all the Opposing Player’s Markers at that Position get ‘bumped’ back to Position 1. Those Markers must start again from Position 1.
• A Player may have more than one of their own Pieces sharing the same Position.
• If the destination of a Player’s chosen Marker EXCEEDS the value of 120 then that Player’s Marker is bumped back to Position 1 and must start again.
• If a Player’s Marker lands exactly on position 120, then that Marker has concluded its journey, and is retired from the game. The Player continues with their remaining Markers.
• The game continues alternating Players until one Player has successfully landed ALL four of their Markers on Position 120.
Design – Algorithm
The algorithm in the code does the following decisions in this order:
Setup
Between Player 1 and Player 2, a starting player is chosen at random.
Scenario Set A – Prioritise advancing on ‘penultimate’ Positions
When a Player rolls a die, the algorithm first looks ahead to see if there is an opportunity to move a Marker to any ‘penultimate’ Positions. Penultimate Positions are those in the number set {20,24,30,40,60} where a single subsequent die roll (respectively: 6,5,4,3,2) will result in the Player landing that Marker exactly at Position 120. If it finds more than one Marker that can target a penultimate Position, it will pick one at random and move it.
Scenario Set B – Prioritise advancing on ‘factor’ Positions
If not, the algorithm goes looking ahead to target a ‘factor number’ which is one of the numbers in the set which, when cumulatively combined with die roles of 2 to 6, could eventually lead to Position 120. (i.e. one possible Marker journey is: 2, 4, 20, 40, 120 based on die rolls of: 2, 2, 5, 2 and 3). In doing this, it increases the likelihood of a Marker fast-tracking it to the end state.
Scenario Set C – Prioritise targeting Opposition Markers
If not, it goes looking to “target” an Opposition Player’s Marker to bump back to Position 1, by applying the product of the die roll value to all active opposition Markers to see which ones it may knock out. (i.e. if Player 1 rolls a 5 and could move a Marker to Position 10, then it goes looking to see if Player 2 has any Markers on Position 10 that it could bump.). The algorithm will pick the first potential target that meets these criteria.
Scenario Set D – Choose a Marker at random
Finally, if it has fallen through these three scenarios conditions, then it will do a coin toss and either:
i) pick a Marker at random which is in play (i.e. on a position > 1) to move, first checking if there is a risk that the Marker could ‘blow out’ by exceeding 120 when applying the die roll value, or
ii) pick a Marker at random that is not yet in play (i.e. on position 1) to move into play.
If all active Markers could potential blow out, then it choses one at random anyway as it must make a move.
End-game Check
After each Marker move, it scans the status of the four Markers for each Player. If a Player has no more Markers in play, they are identified as the winner and the game ends.
NEXT POST… Part 2 – designing the algorithm ‘on paper’ to work through the logic in preparation for turning it into an implementable model for object-orientated coding…